Blog
/
Category
/
Details
১০০+ ফ্লাটার ও ডার্ট টিপস
01 October 2023
•
59 min read
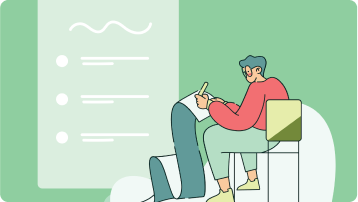
-----লার্নিং//
হ্যালো পাঠক/পাঠিকা,
আমার এই আরর্টিকেলটি লেখার উদ্দেশ্য হচ্ছে ১০০+ টিপস ও ট্রিক্সের একটি তালিকা তৈরি করা। আপনি যদি এই ফ্লাটার/ডার্ট-এ নতুন হয়ে থাকেন, তাহলে এখান থেকে কিছু আপনার কাজে আসতে পারে।
১. entry ব্যবহার করে আপনি নাল-সেইফ (null-safe) পদ্ধতিতে একটা ম্যাপের মধ্য দিয়ে ইটারেট করতে পারেন।
for (var entry in items.entries) {
//print the keys and values
print('${entry.key}: ${entry.value}');
}
২. লিস্টে অনেক ক্ষেত্রে ডুপ্লিকেট আইটেম থাকতে পারে। সুতরাং আপনি যদি একটি ইউনিক ভ্যালুর কালেকশন পেতে চান, আপনি এর পরিবর্তে একটি Set ব্যবহার করুন।
একটি সেটে দুইটি এলেমেন্ট সমান হতে পারে না, তাই নিচের কোডটি কম্পাইল্ড হবে না।
final citiesSet = {'Dublin', 'London', 'Paris', 'Dublin'};
৩. আপনি Future.wait ব্যবহারের মাধ্যমে Futureএর একাধিক ফলাফল সম্পন্ন হওয়ার অপেক্ষা করতে পারেন।
class someAPI {
Future<int> getThings() => Future.value(3000);
Future<int> getItems() => Future.value(300);
Future<int> getStuff() => Future.value(30);
}
...
final api = someAPI();
final values = await Future.wait([
api.getThings(),
api.getItems(),
api.getStuff(),
]);
print(values);
৪. আপনি GridView.count ব্যবহার করে একটি গ্রিড তৈরি করতে পারেন যেটি পোর্ট্রেট মোডে ২ টাইল চওড়া এবং ল্যান্ডস্কেইপ মোডে ৩ টাইল চওড়া।
Flexible(
child: GridView.count(
crossAxisCount: (orientation == Orientation.portrait) ? 2 : 3,
mainAxisSpacing: 4.0,
crossAxisSpacing: 4.0,
padding: const EdgeInsets.all(4.0),
childAspectRatio:
(orientation == Orientation.portrait) ? 1.0 : 1.3,
children: someList
.map(
(catData) => aListItemWidget(catData),
)
.toList(),
),
),
৫. আপনি dart:math থেকে Random ব্যবহার করে একটি লিস্ট থেকে এলোমেলোভাবে অর্থাৎ র্যান্ডমলি যেকোনো আইটেম সিলেক্ট করতে পারেন।
import 'dart:math';
...
int min = 0;
int max = someList.length - 1;
Random rnd = new Random();
int r = min + rnd.nextInt(max - min);
return someList[r];
৬. ডিভাইস ওরিয়েন্টেশন লক করার জন্য আপনি সার্ভিসেস লাইব্রেরি ব্যবহার করতে পারেন।
import 'package:flutter/services.dart';
...
void main() async {
await SystemChrome.setPreferredOrientations([
DeviceOrientation.portraitUp,
]);
runApp(App());
}
৭. যেকোনো প্ল্যাটফর্ম-স্পেসিফিক কোড লিখতে আপনি dart:io library ব্যবহার করতে পারেন।
import 'dart:io' show Platform;
...
if (Platform.isIOS) {
doSomethingforIOS();
}
if (Platform.isAndroid) {
doSomethingforAndroid();
}
৮. যেকোনো কালারকে আপনি একটি ম্যাটেরিয়েল কালারে রূপান্তর করতে পারেন।
const Map<int, Color> color = {
50: Color.fromRGBO(255, 207, 68, .1),
100: Color.fromRGBO(255, 207, 68, .2),
200: Color.fromRGBO(255, 207, 68, .3),
300: Color.fromRGBO(255, 207, 68, .4),
400: Color.fromRGBO(255, 207, 68, .5),
500: Color.fromRGBO(255, 207, 68, .6),
600: Color.fromRGBO(255, 207, 68, .7),
700: Color.fromRGBO(255, 207, 68, .8),
800: Color.fromRGBO(255, 207, 68, .9),
900: Color.fromRGBO(255, 207, 68, 1),
};
const MaterialColor custom_color =
MaterialColor(0xFFFFCF44, color);
৯. স্ট্যাটাস বার হাইড করার জন্য আপনি সার্ভিসেস লাইব্রেরি ব্যবহার করতে পারেন।
import 'package:flutter/services.dart';
void main() {
SystemChrome.setEnabledSystemUIOverlays([]);
}
১০. http প্যাকেজে http রিসোর্স ব্যবহার করার জন্য ফাংশান এবং ক্লাসের একটি সেট রয়েছে। নিচের উদাহরণে দেখা যাচ্ছে কিভাবে http প্যাকেজ ব্যবহার করে http রিকোয়েস্ট করা হয়।
import 'package:http/http.dart' as http;
http.get(some_api_url).then((http.Response res) {
final data = json.decode(res.body);
print(data);
});
১১. আপনি একটি ট্রান্সপারেন্ট প্লেসহোল্ডার ডিসপ্লে করার জন্য FadeInImage উইজেট এবং transparent_image প্যাকেজ ব্যবহার করতে পারেন যাতে করে এটি লোড হতে হতে ইমেজটি ফেইড হওয়ার অপেক্ষায় থাকে।
import 'package:transparent_image/transparent_image.dart';
...
FadeInImage.memoryNetwork(
placeholder: kTransparentImage,
image: someImageUrl,
fit: BoxFit.fill,
),
১২. TextFields-এ আইকন যুক্ত করতে হলে InputDecoration থেকে “icon”, “prefixIcon” এবং “suffixIcon” ব্যবহার করুন।
TextField(
decoration: InputDecoration(
icon: Icon(Icons.email)
),
),
...
TextField(
decoration: InputDecoration(
prefixIcon: Icon(Icons.email)
),
),
...
TextField(
decoration: InputDecoration(
suffixIcon: Icon(Icons.email)
),
),
১৩. TextFields-এর কার্সরটি কাস্টমাইজেবল। নিচের উদাহরণে দেওয়া কোডটি ব্যবহার করলে এটি purple কালারে রূপান্তরিত হবে।
TextField(
cursorColor: Colors.purple,
cursorRadius: Radius.circular(8.0),
cursorWidth: 8.0,
),
১৪. একটা রো’র চিলড্রেনগুলোকে একসাথে কাছাকাছি প্যাক করতে হলে সেটির mainAxisSizeকে MainAxisSize.min এ সেট করুন।
Row(
mainAxisSize: MainAxisSize.min,
children: [
Icon(Icons.star, color: Colors.orange[500]),
Icon(Icons.star, color: Colors.orange[500]),
Icon(Icons.star, color: Colors.orange[500]),
Icon(Icons.star, color: Colors.black),
Icon(Icons.star, color: Colors.black),
],
),
১৫. Expanded widget ব্যবহার করে আপনি একটা উইজেটকে সাইজ করে একটা রো অথবা কলামের ভিতরে ফিট করিয়ে নিতে পারবেন।
Row(
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Expanded(
child: Image.asset('images/pic1.jpg'),
),
Expanded(
child: Image.asset('images/pic2.jpg'),
),
Expanded(
child: Image.asset('images/pic3.jpg'),
),
],
);
১৬. একটা উইজেটকে এক স্ক্রিন থেকে অন্য স্ক্রিনে অ্যানিমেট করার জন্য Hero widget ব্যবহার করুন। উদাহরণস্বরূপ, একটা আইকন উড়ে এক পেজ থেকে অন্য পেজে যাচ্ছে।
// Page 1
Hero(
tag: "FlyingHero",
child: Icon(
Icons.party_mode_outlined,
size: 50.0,
),
),
...
// Page 2
Hero(
tag: "FlyingHero",
child: Icon(
Icons.party_mode_outlined,
size: 150.0,
),
),
১৭. উইজেটগুলোকে ওভারল্যাপ করার জন্য Stack ব্যবহার করুন। একদম উপরের উইজেটটি হবে একদম নিচের উইজেট এবং একদম নিচের উইজেটটি হবে একদম উপরের উইজেট।
Stack(
children: [
Container(
width: 100,
height: 100,
color: Colors.red,
),
Container(
width: 90,
height: 90,
color: Colors.green,
),
Container(
width: 80,
height: 80,
color: Colors.blue,
),
],
)
১৮. আপনার অ্যাপের ভেতরকার সব কালার, ফন্ট, শেইপ আর ডিজাইন স্টাইলের সেটকে ডিফাইন করার জন্য থিম ব্যবহার করুন। এটি এমন এক উপায় যার মাধ্যমে আপনি আপনার সকল স্টাইলিস্টিক ডিসিশনগুলোকে কেন্দ্রীভূত বা সেন্ট্রালাইজ করতে পারবেন।
MaterialApp(
title: appName,
theme: ThemeData(
// The default brightness and colors.
brightness: Brightness.light,
primaryColor: Colors.lightGreen[500],
accentColor: Colors.amber[600],
// The default font family.
fontFamily: 'Georgia',
// TextTheme & text styling
textTheme: TextTheme(
headline1: TextStyle(fontSize: 64.0, fontWeight: FontWeight.bold),
headline6: TextStyle(fontSize: 36.0, fontStyle: FontStyle.italic),
bodyText2: TextStyle(fontSize: 14.0, fontFamily: 'Hind'),
),
),
home: MyHomePage(
title: appName,
),
);
১৯. ডিবাগের ভিতরে কোনো কোড এক্সিকিউট করতে অথবা মোড রিলিজ করতে (package:flutter/foundation.dart)-এর ভেতর থেকে constant (kReleaseMode) ব্যবহার করুন।
import 'package:flutter/foundation.dart';
if (kReleaseMode) {
// Release Mode
print('release mode');
} else {
print('debug mode');
}
২০. একই অবজেক্টের উপরে অপারেশান্স এর সিকোয়েন্স তৈরি করার জন্য Cascade notation ব্যবহার করুন।
List<String> somelist = []
..addAll(['London', 'Dublin', 'Paris'])
..sort()
..forEach((element) => print('\n$element'));
২১. Timer class ব্যবহার করে কিছুক্ষণ পরে একটা কোডের অংশবিশেষ এক্সিকিউট করতে পারেন।
Timer.periodic(
Duration(seconds: 1),
(Timer time) {
print("time: ${time.tick}");
if (time.tick == 10) {
time.cancel();
print('Timer Cancelled');
}
},
);
২২. চাইল্ড উইজেটকে ওভাল অথবা সার্কেল শেইপে ক্লিপ করার জন্য ClipOval উইজেট ব্যবহার করুন। যদি উইড্থ এবং হাইট সমান হয়, তাহলে শেইপটি হবে গোলাকৃতির। আর যদি উইড্থ এবং হাইট আলাদা হয়, তাহলে শেইপটি হবে ওভাল।
ClipOval(
child: Image.network(
imgUrl,
fit: BoxFit.fill,
width: 200.0,
height: 200.0,
),
);
২৩. বাম পাশে এবং ডানপাশে ক্রমানুসারে ক্যারেক্টার প্যাডিং অ্যাড করতে padLeft এবং padRight ব্যবহার করুন।
String s = "fun";
print(s.padLeft(10, ',.*')); // ',.*,.*,.*,.*fun'
print(s.padRight(10, ',.*')); // 'fun,.*,.*,.*,.*'
২৪. একটি স্ট্রিং-এ যেকোনো ধরণের লিস্টে জয়েন করতে হলে List class method join() can ব্যবহার করে জয়েন করতে পারেন।
List<String> values = ['dart', 'flutter', 'fun'];
print(values.join()); // dart,flutter,fun
২৫.ডিবাগ ব্যানার ট্যাগকে রিমুভ করতে হলে debugShowCheckedModeBanner-কে False- এ সেট করুন।
MaterialApp(
debugShowCheckedModeBanner: false,
// other arguments
)
২৬. একটি লিস্টের একটি আইটেমের ইন্ডেক্সে অ্যাক্সেস পেতে হলে asMap ব্যবহার করে লিস্টটিকে একটি ম্যাপে কনভার্ট করে নিতে হবে।
myList.asMap().entries.map((entry) {
int idx = entry.key; // index
String val = entry.value;
return something;
}
২৭. একটা অ্যাপের সবচেয়ে উপরের কম্পোনেন্ট হচ্ছে AppBar ( অথবা মাঝে মাঝে সবচেয়ে নিচের), AppBar- এর লেয়াউটে ৩টি কম্পোনেন্ট থাকেঃ লিডিং, টাইটেল এবং একশান্স।
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(
'App Title',
),
centerTitle: true,
actions: [
IconButton(
icon: Icon(Icons.logout),
onPressed: () {},
),
],
backgroundColor: Colors.yellowAccent[600],
elevation: 50.0,
leading: IconButton(
icon: Icon(Icons.menu),
tooltip: 'Menu Icon',
onPressed: () {},
), //IconButton
brightness: Brightness.dark,
),
body: const Center(
child: Text(
"Enjoy",
style: TextStyle(fontSize: 24),
), //Text
), //Center
); //Scaffold;
}
২৮.যেকোনো ডার্ট প্রোগ্রাম লিখতে হলে, হোক সেটি কোনো স্ক্রিপ্ট অথবা কোনো ফ্লাটার অ্যাপ, আপনাকে অবশ্যই একটি ফাংশানকে ডিফাইন করতে হবে যেটা হলো main()। এটি হচ্ছে সকল অ্যাপের এন্ট্রি পয়েন্ট।
void main() {
var list = ['London', 'Dublin', 'Paris'];
list.forEach((item) {
print('${list.indexOf(item)}: $item');
});
}
২৯. প্রেস করার পরে একটি বাটনের কালার পরিবর্তন করতে পারেন।
TextButton(
onPressed: () {},
style: ButtonStyle(
foregroundColor: MaterialStateProperty.resolveWith<Color>(
(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) return Colors.pink;
return null; // Defer to the widget's default.
}),
),
child: Text(
'Change My Color',
style: TextStyle(fontSize: 30),
),
),
৩০. নিচের উদাহরণটি একটি GetX ডায়লগ ডিসমিস করার পরে একটি কোড রান করা দেখায়।
Get.dialog(MyAlert()).then(
(value) => runSomeCode(),
);
৩১. Enums এবং এক্সটেনশন মেথড কোডকে পড়ার মত পরিচ্ছন্ন করতে পারে। নিচের উদাহরণে আমরা Enums কে Strings এ রূপান্তর করছি।
enum Cities { London, Dublin, Paris }
extension ToString on Cities {
String get name => toString().split('.').last;
}
void main() {
print(Cities.London.name);
print(Cities.Dublin.name);
print(Cities.Paris.name);
}
৩২. ডার্টে আপনি একটি callable ক্লাস বানাতে পারেন যাতে আপনি ফাংশন হিসেবে ক্লাসের একটি ইন্সট্যান্সকে কল করতে পারেন।
class WelcomeToTheCity {
// Defining call method
String call(String a, String b, String c) => 'Welcome to $a$b$c';
}
void main() {
var welcome_to_city = WelcomeToTheCity();
// Calling the class through its instance
var welcome_msg = welcome_to_city('SanDiego ', 'CA ', 'USA');
print(welcome_msg);
}
৩৩. আপনি InkWell ব্যবহার করে একটি ripple effect বানাতে পারেন যা দিয়ে টাচের কাজের জন্য ভিজুয়াল এক্সপেরিয়েন্স তৈরি করতে পারেন।
InkWell(
splashColor: Colors.red,
onTap: () {},
child: Card(
elevation: 5.0,
child: Text(
' Click This ',
style: Theme.of(context).textTheme.headline2,
),
),
),
৩৪. নিচের উদাহরণে আমরা BoxDecoration ও DecorationImage দুটি ব্যবহার করে একটি অ্যাপের ব্যাকগ্রাউন্ড ইমেজ সেট করছি।
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter'),
),
body: Container(
width: double.infinity,
height: double.infinity,
decoration: BoxDecoration(
image: DecorationImage(
image: NetworkImage(image_Url),
),
),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'Title',
style: const TextStyle(
color: Colors.white,
fontSize: 48,
fontWeight: FontWeight.bold),
)
],
),
),
);
}
৩৫. আপনি ListWheelScrollView উইজেট ব্যবহার করে একটি স্ক্রোলেবল হুইলে থ্রিডি এফেক্টসহ ListView বিল্ড করতে পারেন যেন মনে হয় বাচ্চারা একটা হুইলের মধ্যে ঘুরছে।
...
List<Widget> items = [
ListTile(
leading: Icon(Icons.local_airport, size: 50),
title: Text('Airport'),
subtitle: Text('Description'),
),
ListTile(
leading: Icon(Icons.local_convenience_store, size: 50),
title: Text('Convenience Store'),
subtitle: Text('Description'),
),
ListTile(
leading: Icon(Icons.local_dining, size: 50),
title: Text('Dining'),
subtitle: Text('Description'),
),
ListTile(
leading: Icon(Icons.local_drink, size: 50),
title: Text('Drink'),
subtitle: Text('Description'),
),
ListTile(
leading: Icon(Icons.local_florist, size: 50),
title: Text('Florist'),
subtitle: Text('Description'),
),
ListTile(
leading: Icon(Icons.local_gas_station, size: 50),
title: Text('Gas Station'),
subtitle: Text('Description'),
),
ListTile(
leading: Icon(Icons.local_grocery_store, size: 50),
title: Text('Grocery Store'),
subtitle: Text('Description'),
),
];
...
Center(
child: ListWheelScrollView(
itemExtent: 75,
children: items,
)
)
৩৬. আপনি একটি টেক্সটের পেছনে ব্যাকগ্রাউন্ড কালার এবং শুরু ও শেষে একটি কার্ভ যুক্ত করতে পারবেন।
Center(
child: Text(
'This is a very important text ',
style: TextStyle(
fontWeight: FontWeight.w600,
color: Colors.white,
fontSize: 20,
background: Paint()
..strokeWidth = 30.0
..color = Colors.red
..style = PaintingStyle.stroke
..strokeJoin = StrokeJoin.round),
),
)
৩৭. ‘AboutListTile’ নামক রেডিমেড উইজেট দিয়ে একটি অ্যাবাউট বক্স দেখতে পারেন যেটা অ্যাপের অতিরিক্ত ইনফরমেশন যেমন এর ভার্শন, প্রাইভেসি পলিসি, অফিশিয়াল ওয়েবসাইট ইত্যাদি দেখাবে।
....
final List<Widget> aboutBoxChildren = <Widget>[
SizedBox(
height: 20,
),
Text('App information'),
Text('App Privacy Policy'),
Text('App Terms of Service'),
RichText(
text: TextSpan(
children: <TextSpan>[
TextSpan(
style: TextStyle(color: Theme.of(context).accentColor),
text: 'Site URL'),
],
),
)
];
.......
drawer: Drawer(
child: SingleChildScrollView(
child: SafeArea(
child: AboutListTile(
icon: const Icon(Icons.info),
applicationIcon: const FlutterLogo(),
applicationName: 'Amazing About Example',
applicationVersion: 'July 2021',
applicationLegalese: '\u{a9} 2021 The Developer',
aboutBoxChildren: aboutBoxChildren,
),
),
),
),
৩৮. FittedBox ব্যবহার করে চাইল্ড উইজেটকে ভেতরে স্কেল ও ফিট করুন। এটা চাইল্ড উইজেটদের তাদের সাইজ নির্দিষ্ট লিমিটের বাইরে বৃদ্ধিতে বাধা দেয়। এটা তাদেরকে তাদের অ্যাভেইলেবল সাইজে রি-স্কেল করে।
Center(
child: Row(children: [
Expanded(
child: FittedBox(
child: Text(
"It is a long established fact that a reader will be distracted by the readable content of a page when looking at its layout.",
maxLines: 1,
style: TextStyle(fontSize: 23),
),
),
),
]))
৩৯. SafeArea হচ্ছে একটি প্যাডিং উইজেট যা তার চাইল্ডকে যথেষ্ট পরিমাণ প্যাডিং দিয়ে ইনসার্ট করে যাতে করে এটা সিস্টেম স্ট্যাটাস বার, নচ (notches), হোলস (holes), রাউন্ডেড কর্নাস ইত্যাদি থেকে ব্লক পাওয়া থেকে রেয়াই পায়।
@override
Widget build(BuildContext context) {
return Scaffold(
body: SafeArea(
top: true,
bottom: true,
left: true,
right: true,
minimum: EdgeInsets.all(16.0),
maintainBottomViewPadding: true,
child: Text('Check this important text'),
),
);
}
৪০. MediaQuery দিয়ে বর্তমান ডিভাইসের সাইজ, ওরিয়েন্টেশন এবং ইউজার প্রেফারেন্স সম্পর্কে তথ্য পাওয়া যায়।
...
var orientation, size, height, width;
// getting the orientation of the app
orientation = MediaQuery.of(context).orientation;
//size of the widow
size = MediaQuery.of(context).size;
height = size.height;
width = size.width;
...
// checking the orientation
body: orientation == Orientation.portrait
? Container(
color: Colors.green,
height: height / 4,
width: width / 4,
)
: Container(
height: height / 3,
width: width / 3,
color: Colors.yellow,
),
৪১. Mixins এর সহায়তায় আপনি কোডের ব্লক পাগ করতে পারবেন কোনো সাবক্লাস বানানো ছাড়াই।
mixin Fluttering {
void flutter() {
print('fluttering');
}
}
abstract class Bird with Fluttering {
void flap() {
print('flap flap');
}
}
class Hawk extends Bird {
void doHawkThing() {
flap();
flutter();
print('Hawk is Flying');
}
}
main() {
var hawk = new Hawk();
hawk.doHawkThing();
}
৪২. একটি ফাংশনকে আরেকটি ফাংশনে প্যারামিটার হিসেবে পাস করা যায়।
void main() {
const list = ['London', 'Dublin', 'Belfast'];
list.forEach(
(item) => print('City Of $item'));
}
৪৩. Getters ও setters হচ্ছে একটি অবজেক্টের প্রপার্টিতে রিড ও রাইট অ্যাক্সেস দেয়ার বিশেষ মেথড।
class Rectangle {
double left, top, width, height;
Rectangle(this.left, this.top, this.width, this.height);
// Define two calculated properties: right and bottom.
double get right => left + width;
set right(double value) => left = value - width;
double get bottom => top + height;
set bottom(double value) => top = value - height;
}
void main() {
var rect = Rectangle(3, 4, 20, 15);
print(rect.left);
rect.right = 12;
print(rect.left);
}
৪৪. String concatenate বা append করার চারটি ভিন্ন উপায় রয়েছে।
void main() {
//1- Using ‘+’ operator.
String str1 = "Dart";
String str2 = "Is";
String str3 = "Fun";
print(str1 + str2 + str3);
//2- String interpolation.
print('$str1 $str2 $str3');
//3- Directly writing string literals.
print('Dart' 'Is' 'Fun');
//4- Strings.join() method.
// Creating List of strings
List<String> str = ["Dart", "Is", "Fun"];
String _thestr = str.join();
print(_thestr);
_thestr = str.join(" ");
print(_thestr);
}
৪৫. validator উইজেট ব্যবহার করে আপনি আপনার সাবমিট ফাংশনে Form ইনপুট ভ্যালিডেট করতে পারবেন এবং প্রত্যেক TextFormField এ ভ্যালিডেশন কন্ডিশন অ্যাপ্লাই করতে পারবেন।
...
void _submit() {
final isValid = _formKey.currentState.validate();
if (!isValid) {
return;
}
_formKey.currentState.save();
}
...
Form(
key: _formKey,
child: Column(
children: [
Text(
"Form-Validation",
style: TextStyle(fontSize: 24.0, fontWeight: FontWeight.bold),
),
//styling
SizedBox(
height: MediaQuery.of(context).size.width * 0.1,
),
TextFormField(
decoration: InputDecoration(labelText: 'E-Mail'),
keyboardType: TextInputType.emailAddress,
onFieldSubmitted: (value) {
//Validator
},
validator: (value) {
if (value.isEmpty ||
!RegExp(r"^[a-zA-Z0-9.a-zA-Z0-9.!#$%&'*+-/=?^_`{|}~]+@[a-zA-Z0-9]+\.[a-zA-Z]+")
.hasMatch(value)) {
return 'Enter a valid email!';
}
return null;
},
),
//box styling
SizedBox(
height: MediaQuery.of(context).size.width * 0.1,
),
//text input
TextFormField(
decoration: InputDecoration(labelText: 'Password'),
keyboardType: TextInputType.emailAddress,
onFieldSubmitted: (value) {},
obscureText: true,
validator: (value) {
if (value.isEmpty) {
return 'Enter a valid password!';
}
return null;
},
),
SizedBox(
height: MediaQuery.of(context).size.width * 0.1,
),
RaisedButton(
padding: EdgeInsets.symmetric(
vertical: 10.0,
horizontal: 15.0,
),
child: Text(
"Submit",
style: TextStyle(
fontSize: 24.0,
),
),
onPressed: () => _submit(),
)
],
),
),
...
৪৬. ListView দিয়ে scrollDirection প্যারামিটারে Axis.horizontal সেট করে হরাইজন্টাল লিস্ট বানান।
...
ListView(
scrollDirection: Axis.horizontal,
children: [
Container(
height: 480.0,
width: 240.0,
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage('images/image1.png'),
fit: BoxFit.fill,
),
shape: BoxShape.rectangle,
),
),
Container(
height: 480.0,
width: 240.0,
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage('images/image2.webp'),
fit: BoxFit.fill,
),
shape: BoxShape.rectangle,
),
),
Container(
height: 480.0,
width: 240.0,
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage('images/image3.jpg'),
fit: BoxFit.fill,
),
shape: BoxShape.rectangle,
),
),
Container(
height: 480.0,
width: 240.0,
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage('images/image4.jpg'),
fit: BoxFit.fill,
),
shape: BoxShape.rectangle,
),
),
],
),
...
৪৭. floatingActionButtonLocation প্যারামিটারকে নিচের মত করে FloatingActionButton কে মাঝামাঝি আনুন।
...
floatingActionButton: FloatingActionButton(
onPressed: () {},
child: Icon(Icons.settings_voice),
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerFloat,
...
৪৮. আপনি SnackBar যখন এটা প্রেস করা হবে তখন একটা অ্যাকশন লেবেল দিতে পারেন নিচের মত করে।
...
final snackBar = SnackBar(
content: const Text('Amazing SnackBar!'),
action: SnackBarAction(
label: 'Undo',
onPressed: () {
// Do something.
},
),
);
...
৪৯. ইউজার যখন এলার্ট বক্সের বাইরে ট্যাপ করে তখন barrierDismissible প্রপার্টি দিয়ে AlertDialog ডিসমিস হওয়া থেকে প্রতিহত করুন।
...
showDialog<String>(
context: context,
barrierDismissible: false,
builder: (BuildContext context) => AlertDialog(
title: const Text('This is a title'),
content: const Text('This is a description'),
actions: <Widget>[
TextButton(
onPressed: () => Navigator.pop(context, 'Cancel'),
child: const Text('Cancel'),
),
TextButton(
onPressed: () => Navigator.pop(context, 'OK'),
child: const Text('OK'),
),
],
),
),
...
৫০. আপনি ElevatedButton দিয়ে একটি সার্কুলার বাটন বানাতে পারেন। নিচের মত করে।
ElevatedButton(
style: ElevatedButton.styleFrom(
shape: CircleBorder(), padding: EdgeInsets.all(30)),
child: Icon(
Icons.add,
size: 50,
),
onPressed: () {},
)
৫১. ShaderMask উইজেট দিয়ে আপনি একটি গ্রেডিয়েন্ট লুক অ্যাপ্লাই করতে পারেন এবং চাইল্ডে ফিল করাতে পারেন।
body: Center(
child: ShaderMask(
blendMode: BlendMode.srcIn,
shaderCallback: (Rect bounds) {
return LinearGradient(
colors: [Colors.red, Colors.green],
tileMode: TileMode.clamp,
).createShader(bounds);
},
child:
const Text('This is a colorful Text', style: TextStyle(fontSize: 36)),
),
),
৫২. AnimatedSize একটি উইজেট যা অটোম্যাটিকভাবে একটি প্রদত্ত সীমার মধ্যে তার সাইজ ট্রানজিশন করে যখনই প্রদত্ত চাইল্ডের সাইজ পরিবর্তিত হয়।
class _MyStatefulWidgetState extends State<MyStatefulWidget> with TickerProviderStateMixin {
double _size = 50.0;
bool _large = false;
void _updateSize() {
setState(() {
_size = _large ? 250.0 : 100.0;
_large = !_large;
});
}
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: () => _updateSize(),
child: Container(
color: Colors.amberAccent,
child: AnimatedSize(
vsync: this,
curve: Curves.easeInCirc,
duration: const Duration(seconds: 2),
child: FlutterLogo(size: _size),
),
),
);
}
}
৫৩. flutter_staggered_grid_view ব্যবহার করে আপনি বিভিন্ন সাইজের একাধিক কলাম এবং রো’র একটি গ্রিডভিউ তৈরি করতে পারেন।
new StaggeredGridView.countBuilder(
crossAxisCount: 4,
itemCount: 8,
itemBuilder: (BuildContext context, int index) => new Container(
color: Colors.green,
child: new Center(
child: new CircleAvatar(
backgroundColor: Colors.white,
child: new Text('$index'),
),
)),
staggeredTileBuilder: (int index) =>
new StaggeredTile.count(2, index.isEven ? 2 : 1),
mainAxisSpacing: 4.0,
crossAxisSpacing: 4.0,
)
৫৪. আপনি ColorFiltered উইজেট দিয়ে তার চাইল্ড উইজেটে একটি কালার ফিল্টার অ্যাপ্লাই করতে পারেন।
Column(
children: [
// The original image
Image.network(_imageUrl),
// The black-and-white image
ColorFiltered(
colorFilter: ColorFilter.mode(Colors.grey, BlendMode.saturation),
child: Image.network(_imageUrl),
),
],
),
৫৫. flutter_speed_dial প্লাগইন দিয়ে একই স্ক্রিনে একাধিক ফ্লোটিং বাটন বানান।
...
floatingActionButton: SpeedDial(
icon: Icons.share,
backgroundColor: Colors.amber,
children: [
SpeedDialChild(
child: Icon(Icons.face),
label: 'Social Network',
backgroundColor: Colors.amberAccent,
onTap: () {/* Do someting */},
),
SpeedDialChild(
child: Icon(Icons.email),
label: 'Email',
backgroundColor: Colors.amberAccent,
onTap: () {/* Do something */},
),
SpeedDialChild(
child: Icon(Icons.chat),
label: 'Message',
backgroundColor: Colors.amberAccent,
onTap: () {/* Do something */},
),
]),
...
৫৬. একটি টেক্সট উইজেটের জন্য আপনি একাধিক শ্যাডো বানাতে পারবেন। নিচের উদাহরণ দেখুন।
Text('Important Text',
style: TextStyle(
color: Colors.black,
fontSize: 50,
fontWeight: FontWeight.bold,
shadows: [
Shadow(
color: Colors.red[500]!,
offset: Offset(-50, -50),
blurRadius: 5),
Shadow(
color: Colors.green[200]!,
offset: Offset(50, 50),
blurRadius: 5),
],
),
),
৫৭. LayoutBuilder দিয়ে একটি উইজেট ট্রি বানানো যায় যেটা তার প্যারেন্ট উইজেটের উপর নির্ভর করবে।
LayoutBuilder(
builder: (context, constraints) {
if (constraints.maxWidth >= 750) {
return Container(
color: Colors.green,
height: 100,
width: 100,
);
} else {
return Container(
color: Colors.yellow,
height: 100,
width: 100,
);
}
},
);
৫৮. wrap এমন একটি উইজেট যা তার চিল্ড্রেনকে হরাইজন্টাল অথবা ভার্টিকাল রানে ডিসপ্লে করতে পারে। এটা প্রত্যেক চাইল্ডকে মূল অ্যাক্সিসে পূর্ববর্তী চাইল্ডের পাশে রাখতে চেষ্টা করবে। যদি সেখানে যথেষ্ট জায়গা না থাকে, তাহলে এটা আগের চিল্ড্রেনের পাশে ক্রস অ্যাক্সিসে নতুন একটা রান বানাবে।
Wrap(
children: List.generate(
10,
(index) => Container(
margin: const EdgeInsets.all(10),
color: Colors.green,
height: 100,
width: 100,
),
),
);
৫৯. AnimatedIcon হচ্ছে একটা ফ্লাটার উইজেট যা একটা আইকনের সুইচিং আরেকটার সাথে অ্যানিমেট করে।
AnimatedIcon(
icon: AnimatedIcons.pause_play,
size: 52,
progress: myAnimation
),
৬০. AnimatedContainer উইজেট হচ্ছে একটি অ্যানিমেশনসহ কন্টেইনার উইজেট। এর প্রপার্টির ভ্যালু পরিবর্তন করার মাধ্যমে এটাকে অ্যানিমেট করা যাবে।
AnimatedContainer(
width: _width,
height: _height,
decoration: BoxDecoration(
color: _color,
borderRadius: _borderRadius,
),
duration: Duration(seconds: 1),
curve: Curves.fastOutSlowIn,
),
৬১. SliverAppBar দিয়ে একটা ফ্লোটিং অ্যাপ বার আনা যায়।
SliverAppBar(
pinned: _pinned,
snap: _snap,
floating: _floating,
expandedHeight: 160.0,
flexibleSpace: const FlexibleSpaceBar(
title: Text('SliverAppBar'),
background: FlutterLogo(),
),
),
৬২. AnimatedOpacity উইজেট দিয়ে একটা সীমার মধ্যে অটোম্যাটিকভাবে চাইল্ডের অপাসিটি ট্রানজিশন করা যায় যখনই অপাসিটি চেঞ্জ হয়।
AnimatedOpacity(
opacity: _opacity,
duration: const Duration(seconds: 1),
curve: Curves.bounceInOut,
// The green box must be a child of the AnimatedOpacity widget.
child: Container(
width: 200.0,
height: 200.0,
color: Colors.green,
),
),
৬৩. SlideTransition উইজেট দিয়ে এর নরমাল পজিশনের সাপেক্ষে তার পজিশন অ্যানিমেট করা যায়।
SlideTransition(
position: _offsetAnimation,
child: const Padding(
padding: EdgeInsets.all(8.0),
child: FlutterLogo(size: 150.0),
),
)
৬৪. FadeTransition উইজেট অপাসিটি অ্যানিমেশনের মাধ্যমে একটা উইজেটকে ফেড ইন ও আউট করে।
FadeTransition(
opacity: _animation,
child: const Padding(padding: EdgeInsets.all(8),
child: FlutterLogo(),),
),
৬৫. AnimatedCrossFade দিয়ে দুইটি প্রদত্ত চিল্ড্রেনের মাঝে ক্রস-ফেড করা যায় এবং তাদেরকে তাদের সাইজের মধ্যে অ্যানিমেট করা যায়।
AnimatedCrossFade(
crossFadeState: _isFirst
? CrossFadeState.showFirst
: CrossFadeState.showSecond,
duration: const Duration(seconds: 1),
firstCurve: Curves.easeInCubic,
secondCurve: Curves.easeInCirc,
firstChild: const FlutterLogo(
style: FlutterLogoStyle.horizontal, size: 100.0),
secondChild: const FlutterLogo(
style: FlutterLogoStyle.stacked, size: 100.0),
)
৬৬. CupertinoContextMenu উইজেট হচ্ছে একটা ফুল-স্ক্রিন মোডাল রাউট যা চাইল্ডকে লং-প্রেস করলে ওপেন হয়।
CupertinoContextMenu(
child: Container(
color: Colors.red,
),
actions: <Widget>[
CupertinoContextMenuAction(
child: const Text('Action one'),
onPressed: () {
Navigator.pop(context);
},
),
CupertinoContextMenuAction(
child: const Text('Action two'),
onPressed: () {
Navigator.pop(context);
},
),
],
),
৬৭. BackdropFilter উইজেট পূর্বে বিদ্যমান পেইন্টেড কন্টেন্টের উপর একটি ফিল্টার অ্যাপ্লাই করে।
BackdropFilter(
filter: ImageFilter.blur(
sigmaX: 10.0,
sigmaY: 10.0,
),
child: Container(
color: Colors.black.withOpacity(0.2),
),
),
৬৮. RotatedBox তার চাইল্ডকে একটি কোয়ার্টার টার্নের ইন্টিগ্রাল নাম্বারে রোটেট করে।
RotatedBox(
quarterTurns: 2,
child: Text(
'This is a Roated Text ',
style: TextStyle(
fontSize: 55,
fontWeight: FontWeight.bold,
letterSpacing: 0,
),
),
)
৬৯. Spacer উইজেট দিয়ে উইজেটদের মাঝে স্পেস বানানো যায়।
Container(
color: Colors.red,
height: 50,
width: 50,
),
Spacer(),
Container(
color: Colors.green,
height: 50,
width: 50,
),
Spacer(),
৭০. FractionallySizedBox এমন এক উইজেট যা তার চাইল্ডকে মোট বিদ্যমান স্পেসের একাংশতে সাইজ করে।
...
FractionallySizedBox(
widthFactor: 0.5,
heightFactor: 0.25,
child: RaisedButton(
child: Text('Click Me'),
color: Colors.red,
textColor: Colors.white,
onPressed: () {},
),
),
...
৭১. AnimatedSwitcher উইজেট দিয়ে দুইটি উইজেট সুইচ করার সময় অ্যানিমেশন বানান।
...
AnimatedSwitcher(
duration: const Duration(milliseconds: 500),
transitionBuilder: (Widget child, Animation<double> animation) {
return ScaleTransition(child: child, scale: animation);
},
child: Text(
'$_count',
key: ValueKey<int>(_count),
style: Theme.of(context).textTheme.headline4,
),
),
...
৭২. CupertinoAlertDialog দিয়ে আইওএস-স্টাইলের একটি উইজেট বানান, যা ইউজারকে এমন পরিস্থিতি নিয়ে বার্তা দেয় যার ব্যাপারে ব্যবস্থা নেয়া প্রয়োজন।
...
CupertinoAlertDialog(
title: Text("This is the title"),
content: Text("This is the content"),
actions: [
// Close the dialog
// You can use the CupertinoDialogAction widget instead
CupertinoButton(
child: Text('Cancel'),
onPressed: () {
Navigator.of(ctx).pop();
}),
CupertinoButton(
child: Text('I agree'),
onPressed: () {
// Do something
print('I agreed');
// Then close the dialog
Navigator.of(ctx).pop();
},
)
],
)
...
৭৩. CupertinoButton উইজেট দিয়ে একটি আই-ওএস স্টাইলের বাটন বানাতে পারেন যা টাচ করার সাথে সাথে ফেড আউট এবং ফেড ইন হয় এমন একটা টেক্সট বা আইকন নেয়।
...
Container(
child: Center(
child: CupertinoButton(
onPressed: () {
// Todo
},
child: Text("CupertinoButton Widget"),
),
),
),
Container(
child: Center(
child: CupertinoButton.filled(
onPressed: () {},
child: Text("CupertinoButton Widget"),
),
),
),
...
৭৪. CupertinoActionSheet হচ্ছে একটি আইওএস-থিমএড উইজেট যার অ্যাকশন শিট ডিজাইন স্পেসিফিকেশন ( action sheets design specifications) আছে।
...
CupertinoActionSheet(
title: Text(
"Flutter dev",
style: TextStyle(fontSize: 30),
),
message: Text(
"Select any action ",
style: TextStyle(fontSize: 15.0),
),
actions: <Widget>[
CupertinoActionSheetAction(
child: Text("Action 1"),
isDefaultAction: true,
onPressed: () {
print("Action 1 is been clicked");
},
),
CupertinoActionSheetAction(
child: Text("Action 2"),
isDestructiveAction: true,
onPressed: () {
print("Action 2 is been clicked");
},
)
],
cancelButton: CupertinoActionSheetAction(
child: Text("Cancel"),
onPressed: () {
Navigator.pop(context);
},
),
);
...
৭৫. GestureDetector ব্যবহার করে ট্যাপ, ডাবল ট্যাপ, প্রেস, লং প্রেস, প্যান ড্র্যাগ, জুম ইত্যাদি জেসচার ডিটেক্ট করুন।
...
Container(
color: _color,
height: 200.0,
width: 200.0,
child: GestureDetector(
onTap: () {
setState(() {
_color = Colors.yellow;
});
},
),
)
...
৭৬. AnimatedDefaultTextStyle একটি প্রদত্ত ডিউরেশনের মধ্যে ডিফল্ট টেক্সট স্টাইল ট্রানজিশন করে যখনই স্টাইল চেঞ্জ হয়।
...
AnimatedDefaultTextStyle(
duration: Duration(milliseconds: 300),
child: Text("Flutter"),
style: newStyle,
)
...
৭৭. Future.delayed() ব্যবহার করে নির্দিষ্ট সময়ের জন্য প্রোগ্রাম ফ্লো পজ (pause) করুন।
...
await Future.delayed(const Duration(seconds: 1));
...
৭৮. DecoratedBoxTransition ব্যবহার করে DecoratedBox এর বিভিন্ন প্রপার্টিকে অ্যানিমেট করা যায়।
...
DecoratedBoxTransition(
position: DecorationPosition.background,
decoration: decorationTween.animate(_controller),
child: Container(
child: Container(
width: 200,
height: 200,
padding: EdgeInsets.all(20),
child: FlutterLogo(),
),
),
),
...
৭৯. Divider উইজেট ব্যবহার করুন আর একটি ১ পিক্সেল পুরু আনুভূমিক (horizontal) লাইন তৈরি করুন দুইপাশে প্যাডিং (padding) সহ।
...
ListTile(
title: Text('Item1')
),
Divider(),
ListTile(
title: Text('Item2'),
),
...
৮০. DropdownButton উইজেট দিয়ে একটি আইটেম লিস্টে সার্চ করার জন্য একটি বাটন তৈরি করুন।
...
DropdownButton<String>(
value: dropdownValue,
onChanged: (String? newValue) {
setState(() {
dropdownValue = newValue!;
});
},
items: <String>['One', 'Two', 'Free', 'Four']
.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
),
...
৮১. Flow এমন একটি উইজেট যা এর চাইল্ড উইজেটগুলো অ্যারেঞ্জ করে FlowDelegate এর ভিত্তিতে।
...
Flow(
delegate: FlowMenuDelegate(menuAnimation: menuAnimation),
children:
menuItems.map<Widget>((IconData icon) => flowMenuItem(icon)).toList(),
)
...
৮২. Flexible উইজেট দিয়ে Text Overflow এরোর ফিক্স করা যায়।
...
Flexible(
child: Text(
'Flutter Text Overflow while adding long text. how to wrap text in flutter ',
style: TextStyle(fontSize: 20),
),
)
...
৮৩. CheckboxListTile হচ্ছে একটি উইজেট যা একটা চেকবক্স এবং একটি লিস্ট টাইল কম্বাইন করে। এটা দিয়ে আপনি টাইটেল, সাবটাইটেল এবং আইকনসহ একটা চেকবক্স বানাতে পারবেন।
...
CheckboxListTile(
value: _isChecked,
onChanged: (bool value) {
setState(() {
_isChecked = value;
});
},
)
...
৮৪. LinearProgressIndicator উইজেট হচ্ছে একটা ম্যাটেরিয়াল ডিজাইন লিনেয়ার প্রোগ্রেস ইন্ডিকেটর (material design linear progress indicator)।
...
LinearProgressIndicator(
value: controller.value,
semanticsLabel: 'Linear progress indicator',
),
...
৮৫. PageView একটি স্ক্রোলেবল লিস্ট যেটা পেজ বাই পেজ কাজ করে।
...
PageView(
children: <Widget>[
Container(
color: Colors.pink,
child: Center(
child: Text('Page 1'),
),
),
Container(
color: Colors.cyan,
child: Center(
child: Text('Page 2'),
),
),
Container(
color: Colors.deepPurple,
child: Center(
child: Text('Page 3'),
),
),
],
)
...
৮৬. PhysicalModel উইজেটের সাহায্যে আপনি কাস্টম শ্যাডো ইফেক্ট অ্যাড করতে পারবেন এবং এর রঙ ও শেপ কাস্টমাইজ করতে পারবেন।
...
PhysicalModel(
elevation: 6.0,
shape: BoxShape.rectangle,
shadowColor: Colors.black,
color: Colors.white,
child: Container(
height: 120.0,
width: 120.0,
color: Colors.blue[50],
child: FlutterLogo(
size: 60,
),
),
)
...
৮৭. RadioListTile উইজেট হচ্ছে লেবেলসহ একটি রেডিও বাটন।
...
RadioListTile<SingingCharacter>(
title: const Text('Thomas Jefferson'),
value: SingingCharacter.jefferson,
groupValue: _character,
onChanged: (SingingCharacter value) { setState(() { _character = value; }); },
),
...
৮৮. RangeSlider উইজেটটি অনেকগুলো ভ্যালুর একটি রেঞ্জ থেকে একটি রেঞ্জ সিলেক্ট করতে ব্যবহৃত হয়।
...
RangeSlider(
values: _currentRangeValues,
min: 0,
max: 100,
divisions: 5,
labels: RangeLabels(
_currentRangeValues.start.round().toString(),
_currentRangeValues.end.round().toString(),
),
onChanged: (RangeValues values) {
setState(() {
_currentRangeValues = values;
});
},
);
...
৮৯. RefreshIndicator একটি উইজেট যা Material এর swipe-to-refresh সাপোর্ট করে।
...
RefreshIndicator(
onRefresh: () async {
return await Future.delayed(Duration(seconds: 3));
},
child: ListView(
padding: const EdgeInsets.all(8.0),
children: <Widget>[
Container(
height: 50,
color: Colors.amber[600],
child: const Center(child: Text('Entry A')),
),
Container(
height: 50,
color: Colors.amber[500],
child: const Center(child: Text('Entry B')),
),
Container(
height: 50,
color: Colors.amber[100],
child: const Center(child: Text('Entry C')),
),
],
),
),
...
৯০. Switch উইজেট ব্যবহৃত হয় একটি সিঙ্গেল সেটিং টগল অন/অফ করার জন্য।
...
Switch(
value: isSwitched,
onChanged: (value) {
setState(() {
isSwitched = value;
print(isSwitched);
});
},
activeTrackColor: Colors.lightGreenAccent,
activeColor: Colors.green,
),
...
৯১. Tooltip উইজেট একটি বাটনের ফাংশন অথবা other user interface action বিশ্লেষণে সাহায্য করে।
...
Tooltip(
message: 'I am a Tooltip',
child: Text('Hover over the text to show a tooltip.'),
);
...
৯২. SelectableText উইজেট দিয়ে ইউজার ইউআইয়ের কন্টেন্ট সিলেক্ট/কপি করতে পারে।
...
const SelectableText(
'Hello! How are you?',
textAlign: TextAlign.center,
style: TextStyle(fontWeight: FontWeight.bold),
)
...
৯৩. Transform হচ্ছে একটি উইজেট যা নিজের চাইল্ডকে পেইন্ট করার আগে ট্রান্সফরমেশন অ্যাপ্লাই করে।
...
Container(
color: Colors.black,
child: Transform(
alignment: Alignment.topRight,
transform: Matrix4.skewY(0.3)..rotateZ(-math.pi / 12.0),
child: Container(
padding: const EdgeInsets.all(8.0),
color: const Color(0xFFE8581C),
child: const Text('Apartment for rent!'),
),
),
)
...
৯৪. VerticalDivider উইজেট হল একটি ওয়ান ডিভাইস পিক্সেল থিক ভার্টিকাল লাইন (one device pixel thick vertical line), দুইপাশে প্যাডিংসহ।
...
Row(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Container(
child: Text('Item1')
),
VerticalDivider(),
Container(
child: Text('Item2'),
),
VerticalDivider(),
Container(
child: Text('Item3'),
),
],
)
...
৯৫. TimePicker উইজেট একটি ম্যাটেরিয়াল ডিজাইন থিম পিকার সম্বলিত ডায়ালগ শো করে।
...
Future<TimeOfDay> selectedTime = showTimePicker(
initialTime: TimeOfDay.now(),
context: context,
);
...
৯৬. Stepper উইজেট দিয়ে একটি স্টেপের সিকুয়েন্সের মধ্যে প্রগ্রেস ডিসপ্লে করে।
...
Stepper(
steps: [
Step(
title: new Text('Login Info'),
content: Column(
children: <Widget>[
TextFormField(
decoration: InputDecoration(labelText: 'Email Address'),
),
TextFormField(
decoration: InputDecoration(labelText: 'Password'),
),
],
),
isActive: true,
state: StepState.editing
),
],
)
...
৯৭. ActionChip উইজেট দিয়ে চিপস নামক কমপ্যাক্ট এলেমেন্ট বানানো যায়, যা ইউজারের প্রেস করার সাথে সাথে একটা অ্যাকশন ট্রিগার করে।
...
ActionChip(
label: Text('Delete'),
onPressed: () {
print('Processing to delete item');
}
)
...
৯৮. ExpansionPanel হল একটি হেডার ও একটি বডিসহ প্যানেল এবং এটাকে এক্সপ্যান্ড বা কোল্যাপ্স করা যায়। প্যানেলের বডি শুধু তখনই দৃশ্যমান হয় যখন সেটাকে এক্সপ্যান্ড করা হয়।
...
ExpansionPanel(
headerBuilder: (context, isExpanded) {
return ListTile(
title: Text(
'Click To Expand',
style: TextStyle(color: Colors.black),
),
);
},
body: ListTile(
title: Text('Description text',
style: TextStyle(color: Colors.black)),
),
isExpanded: _expanded,
canTapOnHeader: true,
),
...
৯৯. LongPressDraggable উইজেট দিয়ে একটি উইজেট বানানো যায় যেটা লংপ্রেসের মাধ্যমে ড্র্যাগ করা যায়।
...
LongPressDraggable(
feedback: FlutterLogo(
textColor: Colors.orange,
size: 100,
style: FlutterLogoStyle.stacked,
),
child: FlutterLogo(
textColor: Colors.green,
size: 100,
style: FlutterLogoStyle.stacked,
),
onDragEnd: (details) {
setState(() {
final adjustment = MediaQuery.of(context).size.height -
constraints.maxHeight;
_offset = Offset(
details.offset.dx, details.offset.dy - adjustment);
});
},
),
...
১০০. Baseline হল একটি উইজেট যা তার চাইল্ডকে পজিশন করে চাইল্ডের বেজলাইন অনুযায়ী।
...
Center(
child: Container(
color: Colors.blue,
height: 120.0,
width: 120.0,
child: Baseline(
child: Container(
color: Colors.red,
height: 60.0,
width: 60.0,
),
baseline: 20.0,
baselineType: TextBaseline.alphabetic,
),
),
)
...
১০১. Banner উইজেট দিয়ে আরেকটি উইজেটের কোণার উপরে একটি ডায়াগনাল ম্যাসেজ ডিসপ্লে করানো যায়।
...
Center(
child: Container(
margin: const EdgeInsets.all(10.0),
color: Colors.yellow,
height: 100,
child: ClipRect(
child: Banner(
message: "hello",
location: BannerLocation.topEnd,
color: Colors.red,
child: Container(
color: Colors.yellow,
height: 100,
child: Center(
child: Text("Hello, banner!"),
),
),
),
),
),
)
...
১০২. BottomNavigationBar যেটা একটি অ্যাপের নিচে দেখা যায় এবং যেটি দিয়ে কম সংখ্যক ভিউস সিলেক্ট করা যায়, সাধারণত তিন থেকে পাঁচের মধ্যে।
...
bottomNavigationBar: BottomNavigationBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(
icon: Icon(Icons.home),
label: 'Home',
),
BottomNavigationBarItem(
icon: Icon(Icons.business),
label: 'Business',
),
BottomNavigationBarItem(
icon: Icon(Icons.school),
label: 'School',
),
],
currentIndex: _selectedIndex,
selectedItemColor: Colors.amber[800],
onTap: _onItemTapped,
),
...
১০৩. IntrinsicWidth হচ্ছে এমন একটি উইজেট যা তার চাইল্ডকে সাইজ করে চাইল্ডের সর্বোচ্চ অন্তর্নিহিত (intrinsic) প্রস্থে।
...
IntrinsicWidth(
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Container(
width: 200,
height: 100,
color: Colors.teal,
),
Expanded(
child: Container(
width: 100,
height: 100,
color: Colors.red,
),
),
],
),
)
...
১০৪. IntrinsicHeight একটি উইজেট যা তার চাইল্ডকে চাইল্ডের অন্তর্নিহিত (intrinsic) উচ্চতায় সাইজ করে।
...
IntrinsicHeight(
child: Row(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Container(
width: 150,
height: 100,
color: Colors.teal,
),
Container(
width: 150,
height: 200,
color: Colors.red,
)
],
),
)
...
RELATED ARTICLES
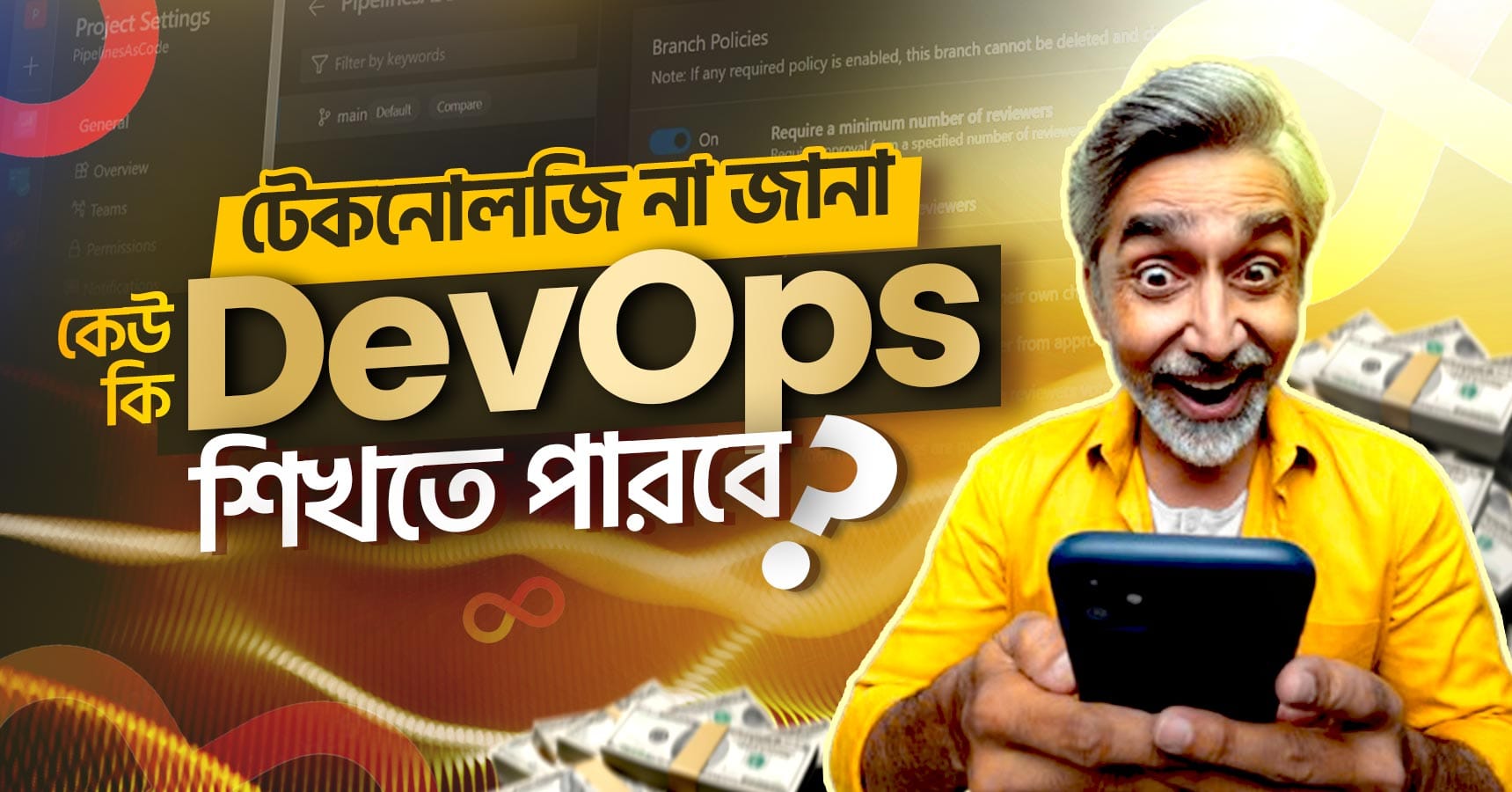
টেকনোলজি না জানা কেউ কি DevOps শিখতে পারবে? || Can A Non_IT Person Learn DevOps? (DevOps Guideline for Non-IT Person)
বর্তমান টেক দুনিয়ায় DevOps (Development and Operations) একটি জনপ্রিয় প্রফেশান হিসেবে গড়ে উঠেছে। DevOps মূলত একটি প্রসেস যা সফটওয়্যার ডেভেলপমেন্ট এবং আইটি অপারেশনস টিমের মধ্যে কম্বাইন করে, যার ফলে ফাস্ট, রিলায়েবল এবং ইফেক্টিভ সফটওয়্যার ডেলিভারি এনশিউর হয়। DevOps-এ ডেভেলপ মানে কেবল টেকনিক্যাল স্কিল নয়, বরং টিম কোলাবোরেশন, কালচারাল চেঞ্জ এবং ডেভেলপ প্রসেসের ইউজের স্কিলও এতে ইনক্লুডেট। আজ আলোচনা করা হবে, Non-IT বা টেকনোলজিক ফিল্ডে না থাকা কেউ কি DevOps শিখতে পারবে? এবং এই প্রফেশানে সাকসেসফুলল
20 October 2024
•
1 min read
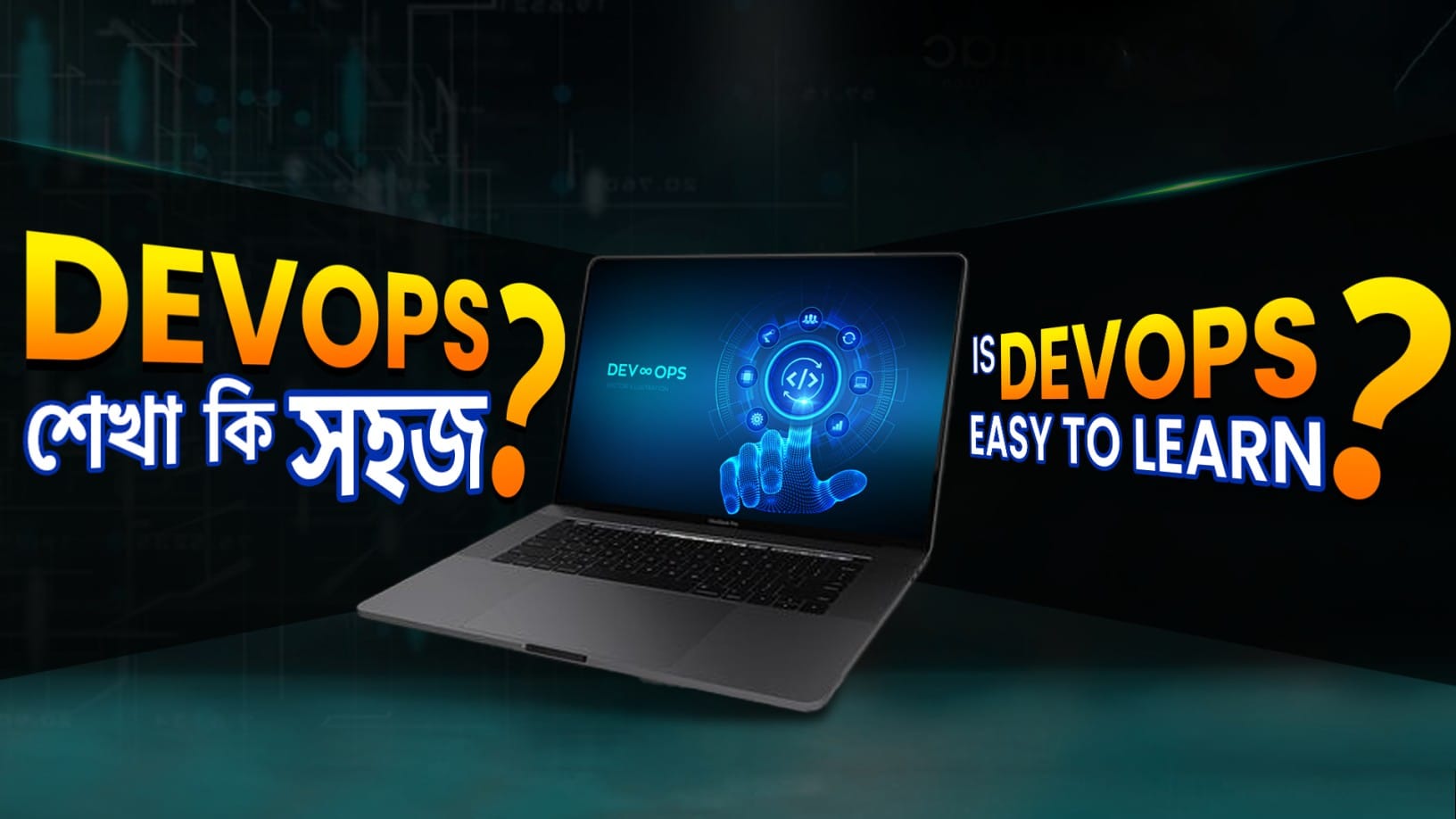
DevOps শেখা কি সহজ? || Is DevOps Easy to Learn? (DevOps Learning Guideline)
বর্তমান টেক দুনিয়ায় DevOps একটি বহুল আলোচিত ও গুরুত্বপূর্ণ আইডিয়া। সফটওয়্যার ডেভেলপমেন্ট ও আইটি অপারেশনের মধ্যে একটি ব্রিজ হিসেবে DevOps-এর ইনোভেশন হয়েছে। এটি সফটওয়্যার ডেভেলমেন্ট ও ডেলিভারির স্পীড বাড়ানোর জন্য অটোমেটিক টুলস ও প্র্যাকটিসের মাধ্যমে কাজ করে। এই DevOps শেখা কি সহজ? এই প্রশ্নের উত্তর অনেকাংশে নির্ভর করে একজন লার্নারের ব্যাকগ্রাউন্ড, এক্সপেরিয়েন্স এবং শেখার ইন্টারেস্টের উপর। আসুন এই বিষয়ে বিস্তারিত আলোচনার আগে আজকের টপিকগুলো জেনে নেই, DevOps কী? DevOps শেখার চ্যালেঞ্জ শেখা
20 October 2024
•
1 min read
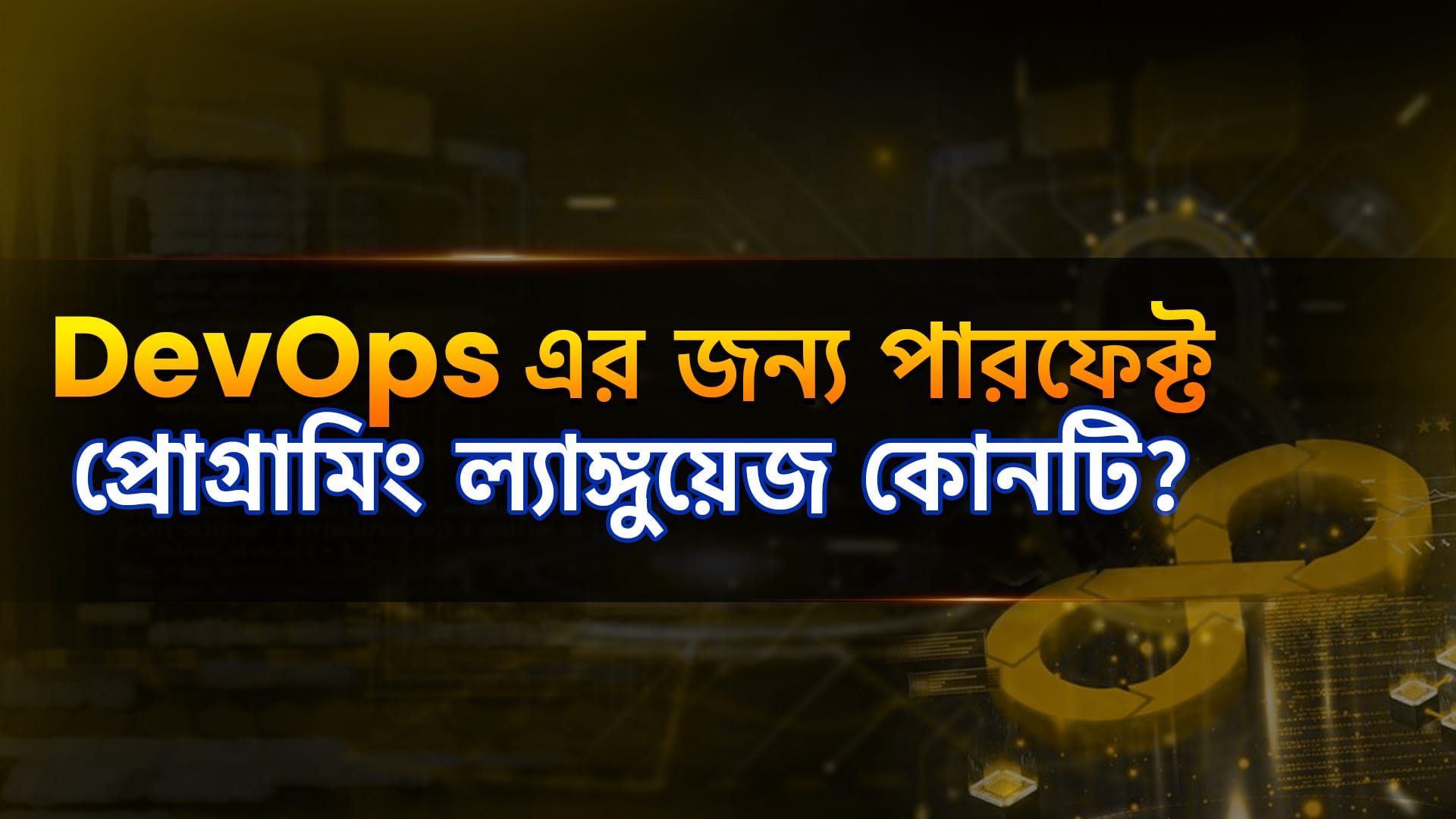
DevOps এর কোন ল্যাঙ্গুয়েজটি বেস্ট? || Which Language is Best for DevOps? || (Best Language For DepOps)
DevOps বর্তমানে সফটওয়্যার ডেভেলপমেন্ট এবং আইটি অপারেশনসের কম্বাইন প্রসেস হিসেবে কাজ করছে। এর মূল উদ্দেশ্য হলো সফটওয়্যার ডেলিভারি প্রসেসকে ফাস্ট এবং কারেক্টলি কমপ্লিট করা, যেখানে ডেভেলপার এবং অপারেশন টিম কোলাবোরেটলি কাজ করে। DevOps প্রসেসে DevOps এর জন্য পারফেক্ট প্রোগ্রামিং ল্যাঙ্গুয়েজের সিলেক্ট করা অত্যন্ত গুরুত্বপূর্ণ কারণ এটি সিস্টেম অটোমেশন, কনফিগারেশন ম্যানেজমেন্ট, ইনফ্রাস্ট্রাকচার ম্যানেজমেন্ট এবং আরও বিভিন্ন কাজে হেল্পফুল হয়। DevOps-এর কাজের প্রসেসে ইউজ করার মতো বিভিন্ন প্রোগ্রামিং ল্
08 October 2024
•
1 min read
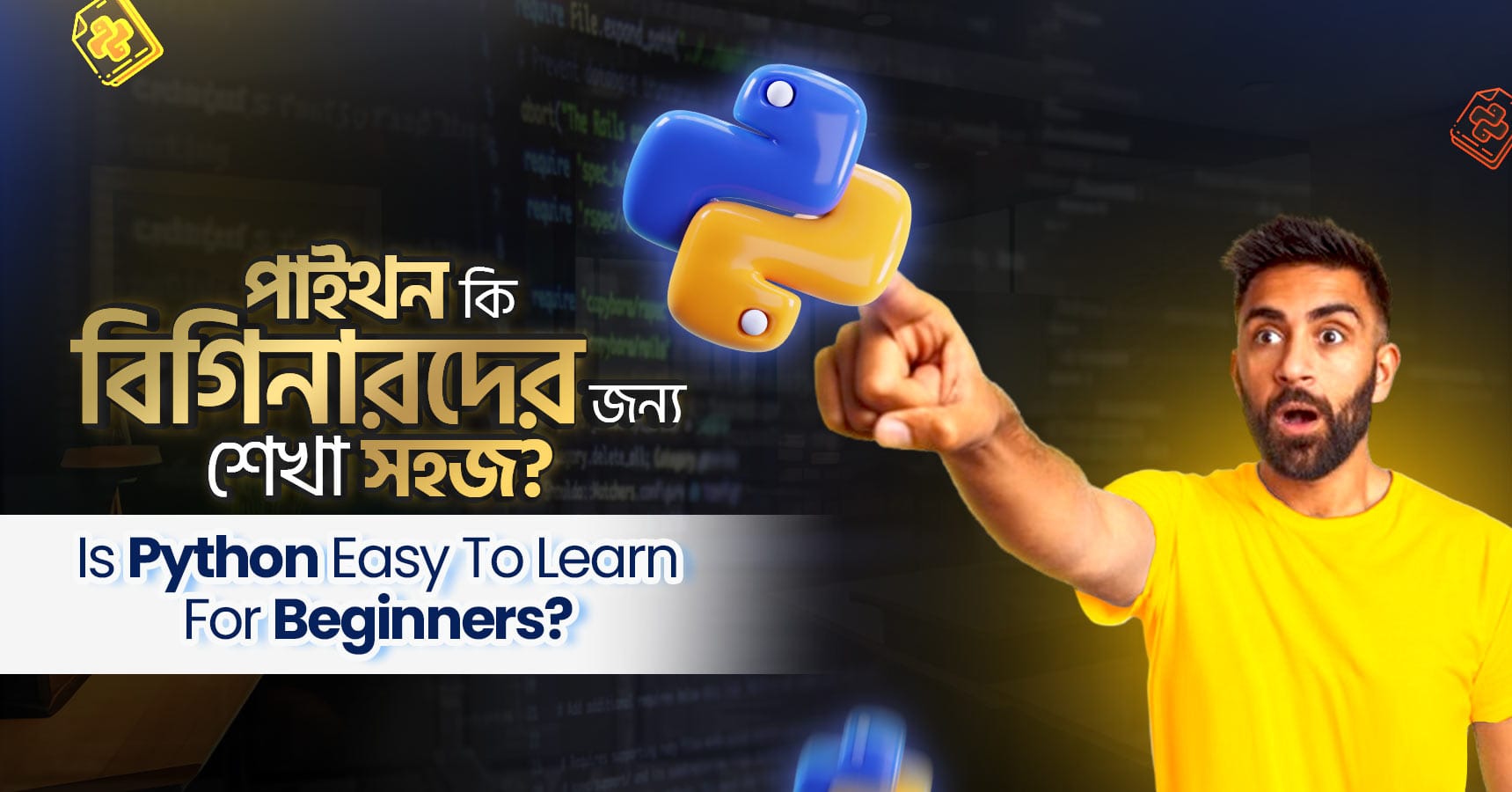
পাইথন কি বিগিনারদের জন্য শেখা সহজ? || Is Python Easy to Learn for Beginners || ( Python Guideline for Beginners)
প্রোগ্রামিংয়ের দুনিয়া বিগিনারদের জন্য অনেকটা কঠিন। প্রোগ্রামিংয়ে আগ্রহ থেকে ক্যারিয়ার হিসেবে অনেকেই প্রোগ্রামার হতে চায়। কিন্তু প্রোগ্রামার হওয়ার জার্নিটা এতো সহজ নয়। দিনের পর দিন কোডিং নিয়ে থাকতে থাকতে অনেকেই হাপিয়ে যায়। ঠিক তখন-ই বিগিনারদের এই কোডিংয়ের ঝামেলা থেকে চলে আসে পাইথন। প্রোগ্রামিং ল্যাঙ্গুয়েজের দুনিয়ায় সবচেয়ে সহজ প্রোগ্রামিং ল্যাঙ্গুয়েজ বলা হয় পাইথনকে। কিন্তু এই কথা কি আসলেই সত্যি? * কেন পাইথন শেখা সহজ? ● পাইথনের ইংরেজি সিনট্যাক্স ● পাইথন লজিকে ফোকাস করে ● পাইথন ফ্রি-তে ইউজ করা
08 October 2024
•
1 min read